Windows PowerShell is a powerful tool for automating tasks and simplifying configuration and can be used to automate almost any task in the Windows ecosystem, including active directory and exchange. It’s no wonder that it’s become a popular tool among sysadmins and experienced Windows users.
In our PowerShell Tutorial, we showed you how to use some of the most useful PowerShell tools. Now it’s time to take the next step: using these tools from within scripts that can be executed with just one click. This PowerShell scripting tutorial will show you how to write and execute basic scripts in PowerShell, and ultimately save you a lot of time.
Get the free PowerShell and Active Directory Video Course
- What is PowerShell ISE?
- PowerShell Uses and Features
- Launching PowerShell
- Basic Features
- Before Running Scripts
- How to Find Commands
- How to Run a Script
- Basic Script Examples
- Windows PowerShell Resources
What is PowerShell Language?
PowerShell language is a high-level proprietary programming syntax developed by Microsoft for the key purpose of enabling system administrators to automate actions and configurations. The language is based on object-oriented standards but can only be used in Windows environments. It is part of the .NET framework and typically has C# code underlying its functions, although knowledge of C# is not a prerequisite for learning PowerShell. The closest comparison to the PowerShell language is Perl, which is used in similar scenarios on Linux environments.
With the PowerShell language, each unique function is referred to as a cmdlet. A cmdlet has one or more sets of defined actions and is capable of returning a .NET object. Some of the most basic cmdlets that come pre-configured with PowerShell are ones for navigating through a folder structure and moving or copying files.
What is the PowerShell ISE?
New PowerShell cmdlet functions can be written in any text editor or word processing tool. However, the latest versions of the Windows operating system include a tool called the PowerShell ISE (Integrated Scripting Environment) to make scripting even easier and more robust.
When you open the PowerShell ISE for the first time, it may look like a familiar command prompt window. However, the tool contains much more functionality and support for writing code. The PowerShell ISE contains a full list of all the common modules and cmdlets that system administrators may need to use. When you are ready to start writing your own cmdlet functions, the debugging tool within the PowerShell ISE will allow you to test your code, identify bugs or issues, and then work to fix them. Like other coding environments, the PowerShell ISE is highly customizable. Users can choose the color scheme, font, and theme they want to use while writing scripts. New scripts created in the ISE will be given the .psi file extension which can only be run in PowerShell environments.
The scripting language in PowerShell will be familiar if you’ve used the Windows Command Prompt. Objects and data piping work in a similar way, for instance, as does ping:
However, the syntax used in PowerShell is, in most instances, much simpler and easier to read than the commands used in Command Prompt.
Windows PowerShell Uses and Features
Though Windows PowerShell can be used for a wide range of different applications, for a beginner, the primary utility of PowerShell scripts will be in regard to systems automation related to:
- Working with batches of files, whether this be to automate backups or to control access to large numbers of files at once.
- PowerShell scripts are also very useful when adding and removing new users. With a carefully designed script, you can automate the process of adding network drives, updating security software, and granting a new user access to shared files.
- In order to perform these tasks, you’ll make use of several key features of PowerShell, such as cmdlets and aliases (which I will cover below).
Launching PowerShell
In Windows 10, the search field is one of the fastest ways to launch PowerShell. From the taskbar, in the search text field, type powershell. Then, click or tap the ‘Windows PowerShell’ result.
To run PowerShell as administrator, right-click (touchscreen users: tap and hold) on the Windows PowerShell search result, then click or tap ‘Run as administrator’.
There are also many other ways to start a PowerShell console, but this is a good method to begin with.
Basic Features of PowerShell
If you are new to PowerShell, take a look at our PowerShell Tutorial before reading this guide to PowerShell scripting. In that guide, you’ll find descriptions of all the basic tools you’ll need to work with PowerShell. This includes cmdlets, aliases, help commands, and pipes.
Once you’ve mastered the basic commands, you can begin to write scripts. As your skills develop, you might also like to take a look at our guides on Input Options for PowerShell, and also read through the resources at the bottom of this article.
Before Running PowerShell Scripts
PowerShell scripts, like those we are going to create in this tutorial, are saved as .ps1 files. By default, Windows will not allow you to run these scripts by just double-clicking the file. This is because malicious (or poorly written) scripts can cause a lot of accidental damage to your system.
Instead, to run a PowerShell script, right-click the .ps1 file, and then click ‘Run with PowerShell’.
If this is your first time working with PowerShell scripts, this might not work. That’s because there is a system-wide policy that prevents execution. Run this command in PowerShell:
Get-ExecutionPolicy
You will see one of the following outputs:
- Restricted— No scripts will be executed. This is the default setting in Windows, so you’ll need to change it.
- AllSigned— You can only run scripts signed by a trusted developer. You will be prompted before running any script.
- RemoteSigned— You can run your own scripts or scripts signed by a trusted developer.
- Unrestricted— You can run any script you want. This option should not be used, for obvious reasons.
To start working with PowerShell scripts, you’ll need to change this policy setting. You should change it to ‘RemoteSigned’, and you can do that right from PowerShell by running the following command:
Set-ExecutionPolicy RemoteSigned
Now you are ready to get started.
How to Find PowerShell Commands
People love PowerShell because it’s so, well, powerful. But that power comes from an absolutely insane amount of complexity. It’s just not feasible or practical for someone to memorize all of the different commands, cmdlets, flags, filters and other ways of telling PowerShell what to do.
Thankfully, built right into the editor are multiple tools to help you deal with this fact.
Tab Completion
There’s no need to memorize all of the different commands or exact spelling of a command. Type get-c into the editor and hit the TAB key – you’ll cycle through all the commands beginning with what you had input so far. This works at any section of the command you’re trying to invoke, the name (as shown below), but also flags and paths that you’re manipulating to get your desired outcome.
Get-Command
While tab completion works well, what happens if you don’t know the name of the command you’re looking for? In that case, you’d use a command for finding other commands:
Get-Command
In searching for commands, it’s important to keep in mind that there’s a syntax to them: VERB-NOUN. Typically the verbs are things like Get, Set, Add, Clear, Write and Read and the Nouns are the files, servers, or other items within your network and applications.
Get-Command is a discovery tool for exploring the commands available on your system.
PowerShell’s Command Syntax
Someone once described the Perl scripting language as looking like “executable line noise” – an incredibly useful tool with a wildly opaque syntax and a correspondingly high learning curve.
While not quite to that level, the traditional command prompt in Windows isn’t too far off. Consider a common task like finding all the items in a directory whose names start with the string ‘Foo’.
CMD: FOR /D /r %G in (“Foo*”) DO @Echo %G
- FOR and DO indicate that it’s a loop.
- The /D flag indicates this is for Directories
- The /r flag indicates that “Files Rooted at Path”
- The pattern that defines the set of files to be looped over is designated with “in”
- @Echo instructs the script to write out the result of each loop and finally;
- %G is the “implicit parameter” and is chosen because earlier developers had already used the pathname format letters a, d, f, n, p, s, t, and x. So, starting with G is traditional as it gives you the largest set of unused letters for returned variables ( G, H, I, J, K, L, M) – in other words, it’s an ugly hack.
Compare that to the PowerShell equivalent:
PowerShell: Get-ChildItem -Path C:\Example -Filter ‘Foo*’
The output’s functionally the same, but even in this fairly trivial example, it’s much, much easier to understand what’s happening. It’s immediately obvious what all the elements in the command do and how you could modify them. The only slightly non-obvious thing here is the * wildcard character (present in both examples) which indicates that the pattern used to match items should start with ‘Foo’ and end in anything else.
It just keeps getting better from here as, say you want to know how to identify just files (not directories) in the path? You could dig up the docs, Google around and try to sort that out with the command line version, or if you’re in PowerShell, type “-” and hit the tab key, rolling through the flag options until the obvious solution shows up.
One Big String vs Object Properties
Servers are no good to anyone if they’re not online. Which is why people spend an inordinate amount of time pretending they’re sonar operators on a submarine and pinging them (yes, that’s actually why it’s called that).
While the output from ping is useful (and you can use ping within PowerShell), at the end of the day the output is just a big string – a series of letter and number characters with no definite breaks between them).
PowerShell has a command that’s analogous to ping, but that returns data that’s structured, making it easy to work with. That command is Test-Connection.
Below you can see the output of pinging a server (named ‘DC’ on their local network) and the equivalent Test-Connection output.
Putting aside that it’s easier to read, what’s really important is that you can now pass this information off to another command, incorporate it into a larger utility (as this full course is working towards) or just tweak it so that it makes more sense.
How To Run A PowerShell Script
There are two main ways to make a PowerShell script:
- The first, which will be familiar if you’ve used Windows Command Line before, is to write scripts directly in notepad. For example, open a new notepad file, and write
Write-Host “Hello World!”
Then save this file as FirstScript.ps1
You can call the script from PowerShell using the command:
& "X:\FirstScript.ps1"
And you’ll see the output in PowerShell.
- The second, much more powerful way of making PowerShell scripts is to use the Windows PowerShell Integrated Scripting Environment (ISE). With ISE, you can run scripts and debug them in a GUI environment.
ISE also features syntax highlighting, multiline editing, tab completion, selective execution, and a whole host of other features. It will even let you open multiple script windows at the same time, which is useful once you have scripts that call other scripts.
Though it might seem like overkill right now, it’s worth working with ISE right from the beginning. That way, you can get used to it before you start writing more complex scripts.
Basic PowerShell Script Examples
Now you can start to write PowerShell scripts. So let’s go through the process step by step.
- Get The Date
- Force Stop Processes
- Check if a File Exists
- Setting Up a VPN
- PowerShell Punctuation Recap
Example Script 1: Get The Date
Let’s start with a simple script. In ISE or notepad, open a new file. Type:
Write-Host get-date
And then save the file as GetDate.ps1
You can call the script from PowerShell using the command:
& "C:\GetDate.ps1"
And you’ll see the output in PowerShell. Simple, right?
Example Script 2: Force Stop A Process
If you have a Windows service running that has frozen, you can use a PowerShell script to stop it. For instance, suppose my company uses Lync for business communications and it keeps freezing and Lync’s process ID is 9212. I can stop Lync with a script.
To do that, make a new script file in the same way as before. This time, type:
stop-process 9212
or
stop-process -processname lync
Save the file as StopLync.ps1, and then you can invoke the script using:
& "X:\StopLync.ps1"
This script can be expanded to stop a number of processes at once, just by adding extra commands of the same type. You can also write another script if you want to automatically start a number of processes at once, using:
start-process -processname [your process here]
This is particularly useful if you want to start a bunch of networking processes at once, for instance, and don’t want to enter the commands separately.
Example Script 3: Check if a File Exists
Suppose you need to delete multiple files, you might want to first check to see if the files even exist.
test-path
, as the name implies, lets you verify whether elements of the path exist. It’ll return TRUE, if all elements exist, and FALSE if any are missing.
You simply type:
test-Path (And then the file path)
Example Script 4: Set up a VPN on a new machine
Now you’ve got the basics, let’s write a script that will actually do something useful. For sysadmins, one of the major advantages of PowerShell is that it allows you to automate the process of setting up new machines.
Today, individuals and businesses alike both use virtual private networks as a near mandatory security measure to protect proprietary data. All new machines should be connected to a VPN during setup. While you could handle each one manually, this is the kind of thing PowerShell is perfect for. For beginners – ie, most people reading this guide – most quality VPN services will work for your computing environment, we can write a script that will automatically set up and configure it.
The most basic way to do this is to open a new file like before, and then type the command:
Set-VpnConnection -Name "Test1" -ServerAddress "10.1.1.2" -PassThru
You will need to set your server address to the address of your local VPN server, and by using the ‘PassThru’ command this script will return the configuration options of the VPN.
Save the file as SetVPN.ps1, and then you should be able to call it in the same way as before, using
& "X:\SetVPN.ps1"
Now. The first time you call this command, you might get some errors. But that’s all part of the process of learning PowerShell scripts. Fear not: whenever you run into an error like this, just take a look at Microsoft’s official guide for the ‘Set-VpnConnection’ command and adapt the examples there to suit your system.
PowerShell Punctuation
Here’s a table to summarize some of the PowerShell punctuation we’ve used:
Symbol | Name | Function | Example |
$ | Dollar Sign | Declares a variable | $a |
= | Equal | Assigns a value to the variable | $a=get-date |
“” | Double Quote | Use double quotes to display text | If $a = Monday
“Day of the Week: $a” Day of the Week: Monday |
+ | Plus | Concatenates | $a = November
“Day of the Week: ” Day of the Week: Monday |
( ) | Parenthesis | Groups to create argument | (get-date).day |
Windows PowerShell Resources
Below are the latest tutorials, and I’ve culled them down to a top ten:
Getting Started with PowerShell
- PowerShell for Beginners – A library of links to get started, best practices, command line syntax and more!
- Don Jones’ bestselling PowerShell book, Learn Windows PowerShell in a Month of Lunches is also in video! After 3-4 months of lunches with the tutorial video series, you’ll be automating admin tasks faster than you ever thought possible. By the way, the author answers questions at powershell.org. There are numerous PowerShell resources, events, and even free ebooks!
- If you’re taking the MCSA 70-410 Microsoft Exam, these flashcards will help: PowerShell commands.
- PowerShell allows you to string multiple commands together on one line using a technique called pipelining. It makes complex things much simpler, and you can learn about it here.
Configure and Manage Active Directory
Save even more time by learning how to configure and manage Active Directory using PowerShell with these resources:
- Use PowerShell to Search AD for High-Privileged Accounts.
- Use AD module cmdlets to perform various administrative, configuration, and diagnostic tasks in your AD DS and AD LDS environments.
- Build an AD utility from scratch in this epic 3 hour PowerShell video course (unlock for free with code: PSHL)
Automate Exchange
With all that free time you have, why not learn how to automate Exchange with these resources:
- If you’re an Exchange Admin, make sure you have these 5 skills down
- Click here for more PowerShell tips for Exchange Admins. Then scroll down for the good stuff.
A Final Word
We hope that this PowerShell scripting tutorial for beginners has given you everything you need to get started with PowerShell scripts. Once you’ve mastered the basics of the PowerShell syntax, working with scripts is pretty easy: just make sure that you keep all your scripts organized, and you name them in a way that it’s obvious what they do. That way, you won’t get confused.
And once you’ve mastered scripts, there really is no end to what you can do with PowerShell. Take a look at our guide to active directory scripting, for instance, for just a taste of the flexibility that PowerShell can provide.
I’ll finish, though, with just one word of warning: don’t rely on PowerShell alone to manage data security and access. Even after you become an expert in PowerShell scripting, the intricacies of GDPR and similar frameworks make data management simply too complex for such a blunt tool. Instead, you should consider consulting an expert on how to manage access to the data stored on your systems.
What should I do now?
Below are three ways you can continue your journey to reduce data risk at your company:
Schedule a demo with us to see Varonis in action. We'll personalize the session to your org's data security needs and answer any questions.
See a sample of our Data Risk Assessment and learn the risks that could be lingering in your environment. Varonis' DRA is completely free and offers a clear path to automated remediation.
Follow us on LinkedIn, YouTube, and X (Twitter) for bite-sized insights on all things data security, including DSPM, threat detection, AI security, and more.
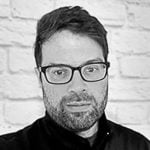